In this short post I will show you what is LogLevel in appsettings.json and what it is used for in ASP.NET Core.
I will try to explain the basics and how it all works so you have stable foundations. If you need a broader understanding of the logging topic in general (and you should if you want to be a good developer) you should definitely check the official Microsoft documentation.
Issue
Most of the time we don’t like spending time on things that are built-in into the framework, like logging, so we tend to skip on fundamentals and take everything for other people built for granted, which usually comes to haunt us at some point into the future.
appsettings.json
When you first create an ASP.NET Core project you will have a file called appsettings.json ( and appsettings.Development.json ) that looks similar to this one

The appsettings.Development.json overrides appsettings.json when we are in Development mode.
This file, as the name suggests, is where you put your app’s settings. By default ASP.NET Core sets pre-defined two categories of logging – Default and Microsoft.AspNetCore.
Default one is pretty much the one you will be using to log and Microsoft.AspNetCore is the one used by the framework (for example when the app starts).
So what does Information and Warning represent? These are logging levels and they tell the framework to log only that level or above.
Here is a table with log levels from the Microsoft docs.
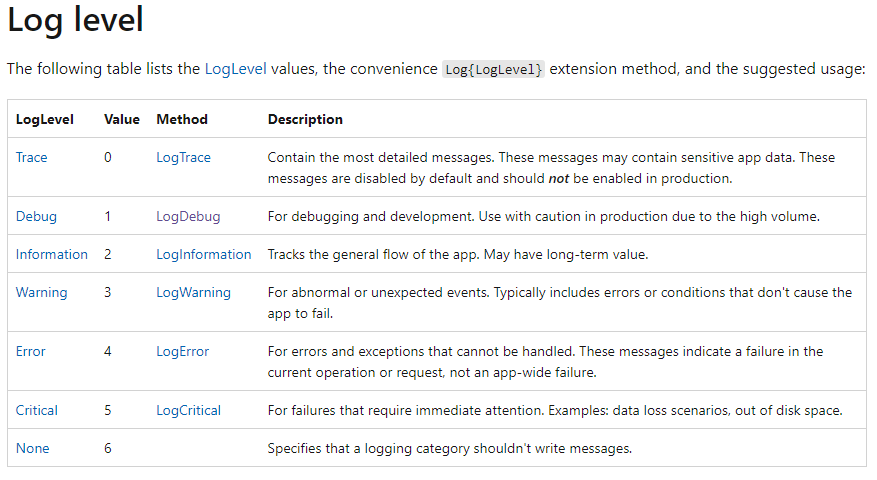
So, the current configuration of logging will log everything that’s Information or above for Default category and everything that’s Warning or above for Microsoft.AspNetCore.
So what does that mean?
Say you have a page called Privacy and in it you have code like that:
public class PrivacyModel : PageModel
{
private readonly ILogger<PrivacyModel> _logger;
public PrivacyModel(ILogger<PrivacyModel> logger)
{
_logger = logger;
}
public void OnGet()
{
_logger.LogDebug("Privacy page visited at", DateTime.UtcNow.ToLongTimeString());
}
}
Given the current appsettings.json configuration the highlighted logging code won’t be executed by the runtime as it’s LogDebug , which is a lower precedence than the Information (see table above).
Also, I should point two things you need to look for when logging like that:
Don’t use string interpolation when logging
_logger.LogDebug($"Privacy page visited at {DateTime.UtcNow.ToLongTimeString()}");
The code above is terrible for performance as it’s creating new string object every time you log, so use the method from before that. Use this method instead.
public static void LogDebug(this ILogger logger, string? message, params object?[] args)
Use logger’s IsEnabled property
Don’t miss that, especially when you have some kind of loop going on that logs on every iteration. Skipping to do that would drain memory as the runtime creates heap objects even when we’re not logging on that level.
Let’s see example
public void OnGet()
{
for (int i = 0; i < 10_000_000; i++)
{
if (_logger.IsEnabled(LogLevel.Debug))
{
_logger.LogDebug($"Privacy page visited at {DateTime.UtcNow.ToLongTimeString()}");
}
}
}
So this should get you started. I see many people not knowing what’s with the logging levels in appsettings.json and the different categories. That’s why I decided to write about this topic in particular. I know it’s short, but I believe it’s a step missed by many, even seasoned developers.
The official Microsoft documentation on logging has a lot of information for you to dig deep, so I suggest you go straight to there from here.
Happy coding.