In this short blog post we’re going to build a simple health check for our app using ASP.NET Core 2.2. What we are going to check against is the availability (readiness) of our SQL Server.
Health checks are exposed by the app as HTTP endpoints, for example, https://example.com/health.
Health checks are divided into two types:
Liveness checks
Basic checks that check the app’s ability to respond to requests.
Readiness checks
More complex checks that check the app’s subsystems and resources availability.
Since we are going to perform a health check on our SQL Server, this health check is a readiness check.
So, let’s begin.
Create an Empty Web App
In Visual Studio: File / New / ASP.NET Core App / Empty
From dotnet CLI: dotnet new web
In the Startup.cs let’s first add the IConfiguration interface so that we’re able to use app’s configuration later.
public IConfiguration Configuration { get; }
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
Now, let’s first create the most basic health check possible.
Add the health check service inside ConfigureServices
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks();
}
Then, let’s insert the service into the request pipeline, inside Configure
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseHealthChecks("/health");
app.Run(async (context) =>
{
await context.Response.WriteAsync("Go to https://localhost:PORT/health.");
});
}
That’s it! We now have a basic health check. Let’s run our app and navigate to /health URI.
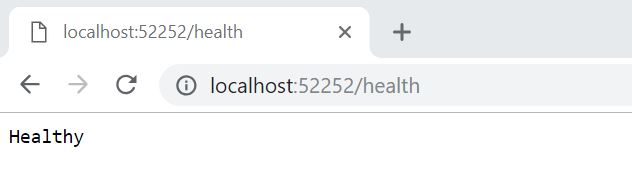
Since this is the most basic health check, all we check is the ability of our app to respond to HTTP requests. That’s why we receive Healthy.
Now, to make things a little bit more interesting, let’s update our health check so that it checks the availability (readiness) of our SQL Server.
To do that we need to install AspNetCore.HealthChecks.SqlServer package. (see more here)
Let’s add a connection string insideappsettings.json
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\mssqllocaldb;Database=invalidDatabase;Trusted_Connection=True;MultipleActiveResultSets=true"
}
The connection string contains invalidDatabase, a database we don’t have. This would make the health check return unhealthy.
We only have to change our ConfigureService and we are done.
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks()
.AddSqlServer(Configuration["ConnectionStrings:DefaultConnection"]);
}
That’s it. If we now run our app again and go to /health URI we will get Unhealthy.
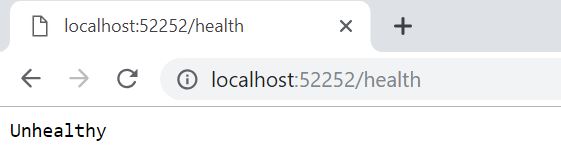
It is that easy to get up and running.
If you need to learn more about health checks in ASP.NET Core visit Microsoft’s documentation by clicking here.
If you want to try health checks against some other provider, please visit this github repo.
Thank you and happy coding!
Absolutely great blog here. This blog has quite in-depth information regarding the health checks with asp.Net core